Advanced Typescript
Learning notes from a few advanced topics in typescript
Rajat Vijay
Frontend Engineering Manager

Certa - No Code workflow management and automation (SAAS)
Typescript
ReactJS

@rajatvijay

@rajatvijay
Agenda
Type Guards
Leveraging type safety for the data fetched at runtime
Testing Types
Treating types as code
Idiot proof switch statements
Never miss a case to handle ever
Type Guards
A type guard is some expression that performs a runtime check that guarantees the type in some scope.
-- TS Docs
let data:any;
// Inferred type is any
data;
if (typeof data === "number") {
// Inferred type is number
data;
}
function fetchCount() {
return axios
.get("API_URL")
.then((response) => response.data)
.then((response) => {
// Inferred type as any
response;
assertIsNumber(response);
// Inferred type as number
return response;
});
}
// Initially
fetchCount: () => Promise<any>
// After using type guards
fetchCount: () => Promise<number>
Actual Use Case
type Employee = {
id: string;
employee_name: string;
employee_salary: string;
employee_age: string;
profile_image: string;
};
export const getEmployees = async () => {
const response = await axios.get(
"http://dummy.restapiexample.com/api/v1/employees"
);
const responseFromAPI = response.data;
// Inferred type is any
responseFromAPI;
assertIsEmployeeArray(responseFromAPI);
// Inferred type is Employee[]
responseFromAPI;
return responseFromAPI;
};
How to write user defined type guards?
Implement `assertIsEmployeeArray`
Other examples of type guards
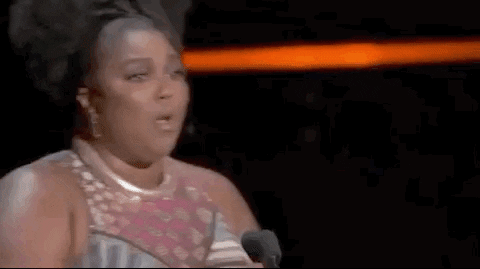
typeof / instanceOf / isArray
function func(value: Function|Date|number[]) {
if (typeof value === 'function') {
// Inferred typs is Function
value;
}
if (value instanceof Date) {
// Inferred typs is Date
value;
}
if (Array.isArray(value)) {
// Inferred typs is number[]
value;
}
}
Tests for types!
Types are also code
They change and grow over time
Jest is not enough
Jest cannot test the changes in your time, it only tests the compiled code
Solution?
TSD to the rescue
Idiot proof swithces
Switches are considered anti pattern
Still there are valid use cases for them!
Super easy to miss a case
Usually used for large no of cases
Solution?
Typescript's `never` type
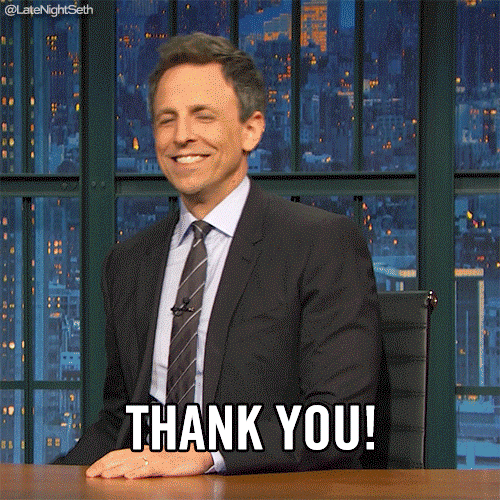

@rajatvijay

@rajatvijay
Advanced Typescript
By rajat vijay
Advanced Typescript
Learning notes from a few advanced topics in typescript. Type Guards, Tests for types, Idiot proof switch statements
- 230